After going through a few ideas, I decided to make my own version of Dance Dance Revolution. With swords. Sword Sword Revolution. Played by swinging an actual sword. This is going to be awesome.
So, the first problem to address: what programming language should I use? I have created games in the past in a few different programming languages, notably Java, Python, and VB.NET, and I thought of all of those, as well as giving PHP a try since that is what I program in professionally. I had originally decided on Python, but after some research and a few sample programs, I decided against it. Pygame is great for working with sprites, but an artist I am not. Instead, I had found some animated GIFs to cover all possible actions for my game, and unfortunately, Pygame can not, to the best of my knowledge, display animated GIFs. At least not simply and natively. Java, on the other hand, can display them just fine inside of an ImageIcon object, so that is what I ultimately chose.
Now, I have not touched Java in over a year, so I knew this project could be a bit of a challenge. That is why I started out with the basics. I knew I wanted a general game screen, with a place to display the animation and a place to show the commands, i.e. which button to press. Since this is pretty basic, I decided to use a JPanel with BorderLayout.
Creating just a basic Java Class, I needed to create the JPanel, a JFrame to hold the JPanel, load the images, and store the required image in a JLabel, which would be displayed in the JPanel. So, basically, JFrame > JPanel > JLabel > ImageIcon.
import java.awt.BorderLayout; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; public class GameWindow extends JPanel{ //Create the necessary variables to be used throughout the game private JLabel mainWindow; private ImageIcon forward; private ImageIcon backward; private ImageIcon left; private ImageIcon right; private ImageIcon ready; public GameWindow(){ //Load the images forward = new ImageIcon("src/images/forward.gif"); backward = new ImageIcon("src/images/backward.gif"); left = new ImageIcon("src/images/left.gif"); right = new ImageIcon("src/images/right.gif"); ready = new ImageIcon("src/images/ready.gif"); //Create a JLabel with the initial image mainWindow = new JLabel(ready); this.setSize(600,600); this.setLayout(new BorderLayout()); //Add the JLabel with image. This will be the main focus of the window, so use the CENTER of the BorderLayout this.add(mainWindow, BorderLayout.CENTER); } public static void main(String[] args) { //Instantiate our game window on initial run GameWindow g = new GameWindow(); //We need a frame to put the panel in JFrame frame = new JFrame("Sword Sword Revolution"); frame.add(g); frame.setSize(600,600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
And with that, I had the basic beginnings of my game. Sure, for now all that it did was display an animated gif, but soon, soon it would do so much more.
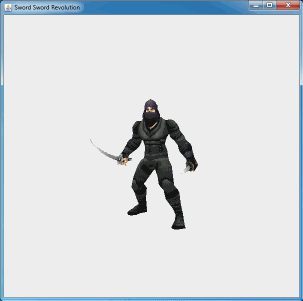
Next, there are four major things that need to happen.
1) I need to change the image in the JLabel when an arrow key is pressed. After a set amount of time, I need to revert the image back to the starting image.
2) I need to add a component to the SOUTH section (or perhaps EAST or WEST) to hold the arrow commands.
3) I need to randomly generate the arrows that come through, and animate them across their box.
4) I need to add a listener that will determine if the user pressed the correct arrow key or not, and the game should react accordingly.
There are also some optional things that would be nice to add:
1) It would be cool if there were enemies animated on the screen. Perhaps coming from the direction you were supposed to swing the sword from. This might be rather challenging, especially since I have the sword swinging left, right, forward, and backward, but would be really neat if I could figure it out.
2) If the player misses a key press or presses incorrectly, I would like a different animation to play.
3) Some background music could be a nice addition.
No comments:
Post a Comment